In this post, we’re going to discuss how to scrape one of the most important features for building a successful NBA prop bets model. That feature is potential assists. And it’s specifically helpful for building an over/under assists model.
First, let’s discuss what a potential assist actually is. A potential assist is a pass that leads directly to a shot, foul or turnover. If you watch basketball, you’ve probably noticed that the number of assists a player gets is largely dependent on how good his teammates are shooting that night. Let’s put it this way, consider two scenarios: In scenario one, player 1 makes three passes to player 2, and all three times player 2 shoots and makes a three pointer. That goes for both three potential assists and three assists for player 1. Each potential assist was actually realized as an actual assist, because player 2 made all his three 3-point attempts on passes from player 1. Now let’s consider scenario 2 – player 1 passes ten times to player 2, and player 2 shoots ten threes, making only two. This counts for TEN potential assists for player 1, while only two actual assists.
Now comes the important question, which scenario do you prefer? The answer is, it’s complicated, but in the long run, scenario 2 is definitely preferable to scenario 1. No one in the history of basketball is a 100% three point shooter, so we can’t expect 3 potential assists to translate to 3 assists too often. On the contrary, most NBA players don’t shoot 20% from the field, so we can’t expect 10 potential assists to translate to 2 assists often – usually more like 3 or 4 assists. This is why just knowing the number of assists a player had in a game is a misleading statistic. By using potential assists as a feature, you can take into account how repeatable his previous assist totals are going forward.
With that being said, let’s dig into the code. You’ll need the NBA API for this, and if you don’t have in installed yet, you can check out our previous blog post on working with the NBA API.
import pandas as pd
from nba_api.stats.endpoints import leaguedashptstats
Below we’ve created an easy to use function for you to scrape potential assists in addition to two other useful columns, passes made and passes received. The function itself takes in season, season_type, and game_date as arguments, and returns the three aformentioned features for all the players that played on that game date.
The parameters that the leaguedashptstats endpoint takes in as arguments are predefined, so just copy and paste the function exactly as is below.
def get_passing_stats(season, season_type, game_date):
player_dash_info = leaguedashptstats.LeagueDashPtStats(
last_n_games = 0,
pt_measure_type = "Passing",
player_or_team = "Player",
per_mode_simple = "PerGame",
season = season,
season_type_all_star = season_type,
team_id_nullable = 0,
opponent_team_id = 0,
date_from_nullable = game_date,
date_to_nullable = game_date
)
player_logs = player_dash_info.get_data_frames()[0]
player_logs["GAME_DATE"] = game_date
player_logs["GAME_DATE"] = pd.to_datetime(player_logs["GAME_DATE"])
return player_logs
And there you have it! Below is a dataframe the tells you how many passes made, passes received, and potential assists a player had for any particular game date.
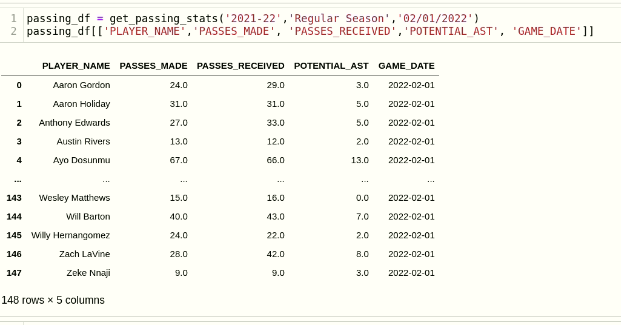